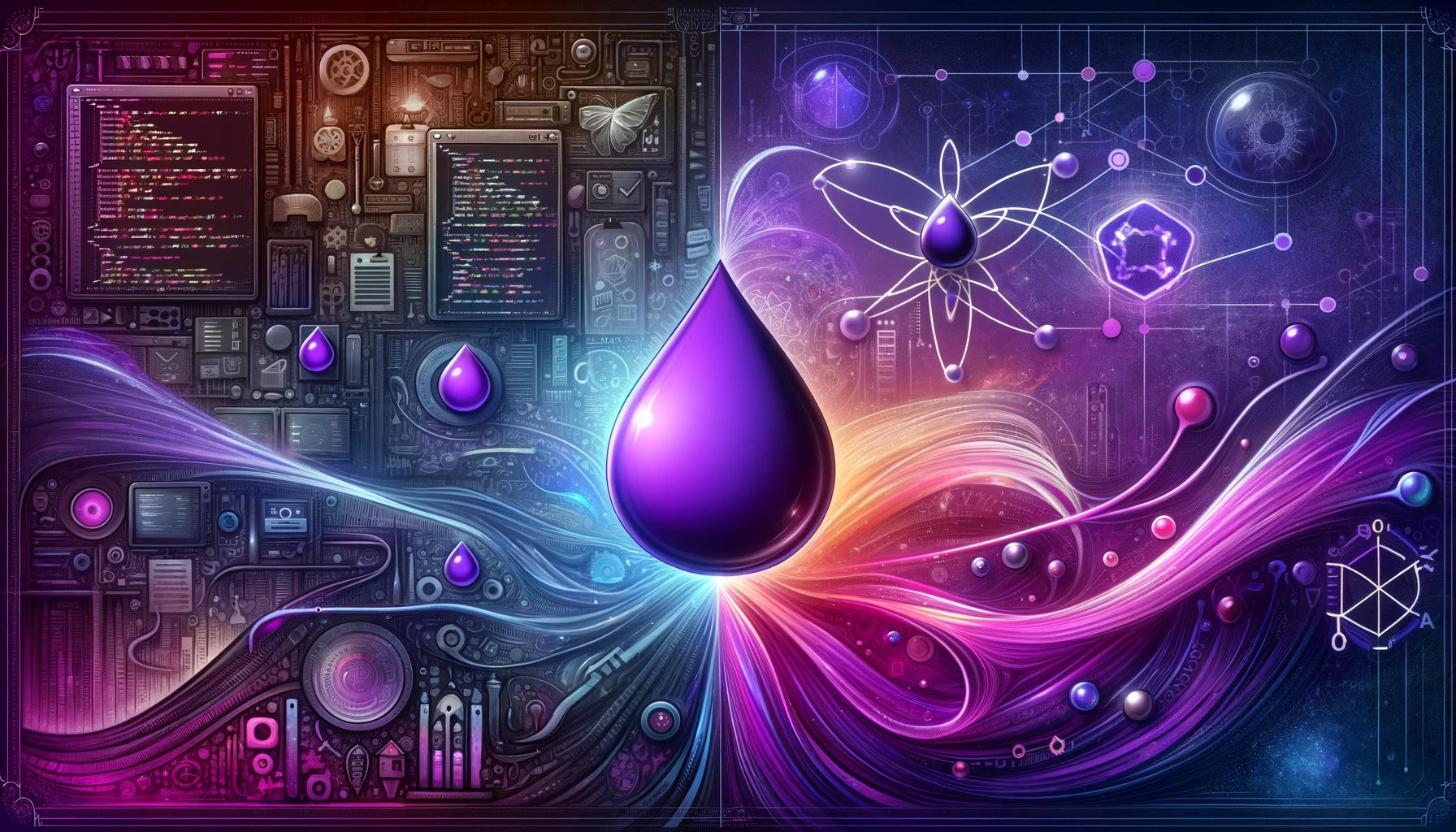
Elixir + Phoenix: My Journey into Functional Web Development
I’m learning functional programming through Elixir and the Phoenix web framework. I’m having a blast. There’s much to love, so let’s dive into some early experiences with Elixir + Phoenix.
I fell in love with pattern matching
It’s still early days in my journey, so I don’t have that much to say about Elixir yet. What I knew before starting this journey, is that pattern matching is quite the thing in functional programming languages. I understand why. After just a few hours I already fell in love with it. It’s really good. I’m no expert on pattern matching yet, but let me give you a simple example. Let’s say we have a function that can print "Hello, <name>!"
on the screen:
def say_hello(name) do IO.puts "Hello, #{name}!"end
What happens if we call this function with a nil
value? It prints "Hello, !"
That’s not what we want. Let’s create a fallback for when no name is provided.
def say_hello(nil) do IO.puts "Hello, you!"end
def say_hello(name) do IO.puts "Hello, #{name}!"end
Through the power of pattern matching, a nil
value matches the first implementation of the say_hello
function by matching the nil
argument. A string value would match the second one.
Another example of pattern matching is when you have map
with named values and want to store a value from that map in a variable:
def get_first_name do person = %{first_name: "John", last_name: "Doe", age: 42}
%{first_name: first_name} = person
IO.puts first_name # Outputs: Johnend
These are just some simple examples, but I already see how powerful this is.
Phoenix provides an amazing developer experience
Further more, Phoenix has been a joy to work with. It’s kind of similar to Laravels approach. It offers a lot of functionality out of the box through the mix
command (which is Elixirs package manager and task runner). If you know Laravel, you can compare the mix
commands to the php artisan
commands.
The mix phx.gen
command allows you to generate files. To create a schema and migration, you can use mix phx.gen.schema
, an HTML resource is created through mix php.gen.html
and so on. It really helps speeding up development.
Not fully comfortable yet
Even though I really like Elixir and Phoenix, I’m not quite comfortable with it yet. It’s very different from when I learned Go. I felt productive in just a few hours. Elixir takes a little longer to feel comfortable with. That probably has more to do with the shift towards functional programming, than with the Elixir language.
I’ll fiddle around some more. Maybe in a few weeks or months I’ll post an update again to see how I’ve progressed.